20.5 Object Serialization
Object serialization allows the state of an object to be transformed into a sequence of bytes that can be converted back into a copy of the object (called deserialization). After deserialization, the object has the same state as it had when it was serialized, barring any data members that were not serializable. This mechanism is generally known as persistence—the serialized result of an object can be stored in a repository from which it can be later retrieved.
Java provides the object serialization facility through the ObjectInput and Object-Output interfaces, which allow the writing and reading of objects to and from I/O streams. These two interfaces extend the DataInput and DataOutput interfaces, respectively (Figure 20.1, p. 1235).
The ObjectOutputStream class and the ObjectInputStream class implement the Object-Output interface and the ObjectInput interface, respectively, providing methods to write and read binary representation of both objects as well as Java primitive values. Figure 20.9 gives an overview of how these classes can be chained to underlying streams and some selected methods they provide. The figure does not show the methods inherited from the abstract OutputStream and InputStream superclasses.
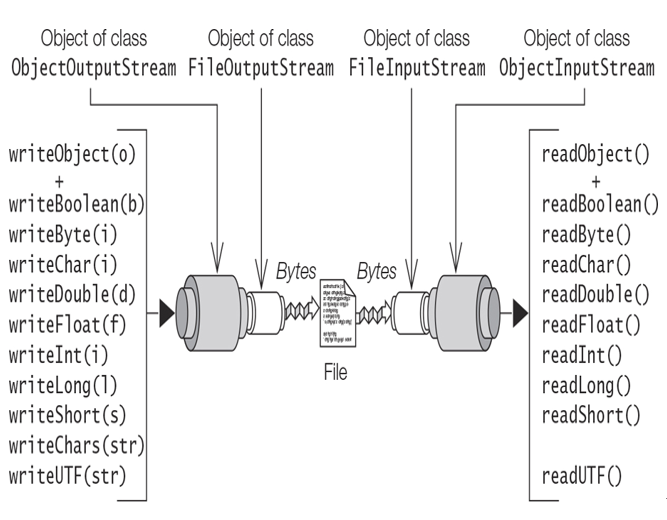
Figure 20.9 Object Stream Chaining
The read and write methods in the two classes can throw an IOException, and the read methods will throw an EOFException if an attempt is made to read past the end of the stream.
The ObjectOutputStream Class
The class ObjectOutputStream can write objects to any stream that is a subclass of the OutputStream—for example, to a file or a network connection (socket). An Object-OutputStream must be chained to an OutputStream using the following constructor:
ObjectOutputStream(OutputStream out) throws IOException
For example, in order to store objects in a file and thus provide persistent storage for objects, an ObjectOutputStream can be chained to a FileOutputStream:
FileOutputStream outputFile = new FileOutputStream(“obj-storage.dat”);
ObjectOutputStream outputStream = new ObjectOutputStream(outputFile);
Objects can be written to the stream using the writeObject() method of the Object-OutputStream class:
final void writeObject(Object obj) throws IOException
The writeObject() method can be used to write any object to a stream, including strings and arrays, as long as the object implements the java.io.Serializable interface, which is a marker interface with no methods. The String class, the primitive wrapper classes, and all array types implement the Serializable interface. A serializable object can be any compound object containing references to other objects, and all constituent objects that are serializable are serialized recursively when the compound object is written out. This is true even if there are cyclic references between the objects. Each object is written out only once during serialization. The following information is included when an object is serialized:
- The class information needed to reconstruct the object
- The values of all serializable non-transient and non-static members, including those that are inherited
A checked exception of the type java.io.NotSerializableException is thrown if a non-serializable object is encountered during the serialization process. Note also that objects of subclasses that extend a serializable class are always serializable.